Table Of Content

The next section will explore the Adapter and Bridge patterns in more detail. Great passion for accessible education and promotion of reason, science, humanism, and progress. Another important thing to mention is the uniformity and universality. Instead of explaining a common concept to someone else, you can simply tell them to utilize a certain pattern.
Design patterns
Yet, it breaks the open-closed principle, as modifying existing classes is mandatory whenever a new operation emerges. Unlike other creational patterns, Builder doesn’t require products to have a common interface. That makes it possible to produce different products using the same construction process. Your objects relate to each other, but for a specific operating system, you are going to create special objects for them. Hiding the object creation, we can have different factories, and based on the operating system we get a specific factory with factory interface.
4 Prototype Method
9 Clean Code Patterns I wish I knew earlier by Martin Thoma - Towards Data Science
9 Clean Code Patterns I wish I knew earlier by Martin Thoma.
Posted: Sat, 16 Oct 2021 07:00:00 GMT [source]
They provide a standardized way to approach these problems, making it easier for developers to create efficient and maintainable code. In this guide, we'll explore some of the most common design patterns in Java, complete with examples and code snippets. Design patterns are reusable solutions to common software design problems. They offer a structured approach to solving issues in software development and are not specific to any particular programming language.
Creational Design Patterns
You had to rely on class components or techniques like render props and higher-order components (HOCs) to achieve stateful behavior. Hooks were introduced to revolutionize this by allowing you to “hook into” React state and lifecycle features from functional components. This simplified development, improved code readability, and made functional components a more viable choice for complex state management. So in behavioral design we are trying to deal with algorithms and the assignment of responsibilities between objects. Patterns are a toolkit of solutions to commonproblems in software design. They definea common language that helps your teamcommunicate more efficiently.
The Facade class has instances of all three subsystems and provides a performOperation() method, which calls the operation methods of all subsystems. Clients interact with the Facade class, which simplifies the interaction with the subsystems. The Composite pattern allows treating a group of objects as a single instance of an object. It composes objects into tree structures to represent part-whole hierarchies. The Circle class (refined abstraction) extends Shape and overrides the draw() method, delegating the call to the Renderer member.
Behavioural Software Design Patterns in Java
In Java, design patterns help maintain code modularity and promote good coding practices. Design patterns are essential tools for writing clean, maintainable, and scalable Java code. They provide solutions to common software design problems and promote good coding practices. By incorporating design patterns into your programming toolkit, you can streamline development and enhance the quality and maintainability of your Java applications. Implementing design patterns in Java requires an understanding of the patterns themselves, as well as a familiarity with Java syntax and programming concepts. In this guide, we will provide an overview of some commonly used design patterns in Java and show you how to implement them in your code.
Mastering Essential Design Patterns for Java Development Success
The implementation of the bridge design pattern follows the notion of preferring composition over inheritance. The composite pattern is used when we have to represent a part-whole hierarchy. When we need to create a structure in a way that the objects in the structure have to be treated the same way, we can apply the composite design pattern. A design patterns are well-proved solution for solving the specific problem/task. A design pattern is a generic repeatable solution to a frequently occurring problem in software design that is used in software engineering.
Top Behavioral Design Patterns With Real Examples In Java
There shall be a separate concrete class per possible state of an object. Each concrete state object will have logic to accept or reject a state transition request based on its present state. A use case can be when we have complex data structure(s), and we want to hide the implementation and complexity of traversing the elements. This pattern is very good when we need to add or remove chains easily. Also, you can set the order for the methods and chains to do one by one.
Builder Pattern
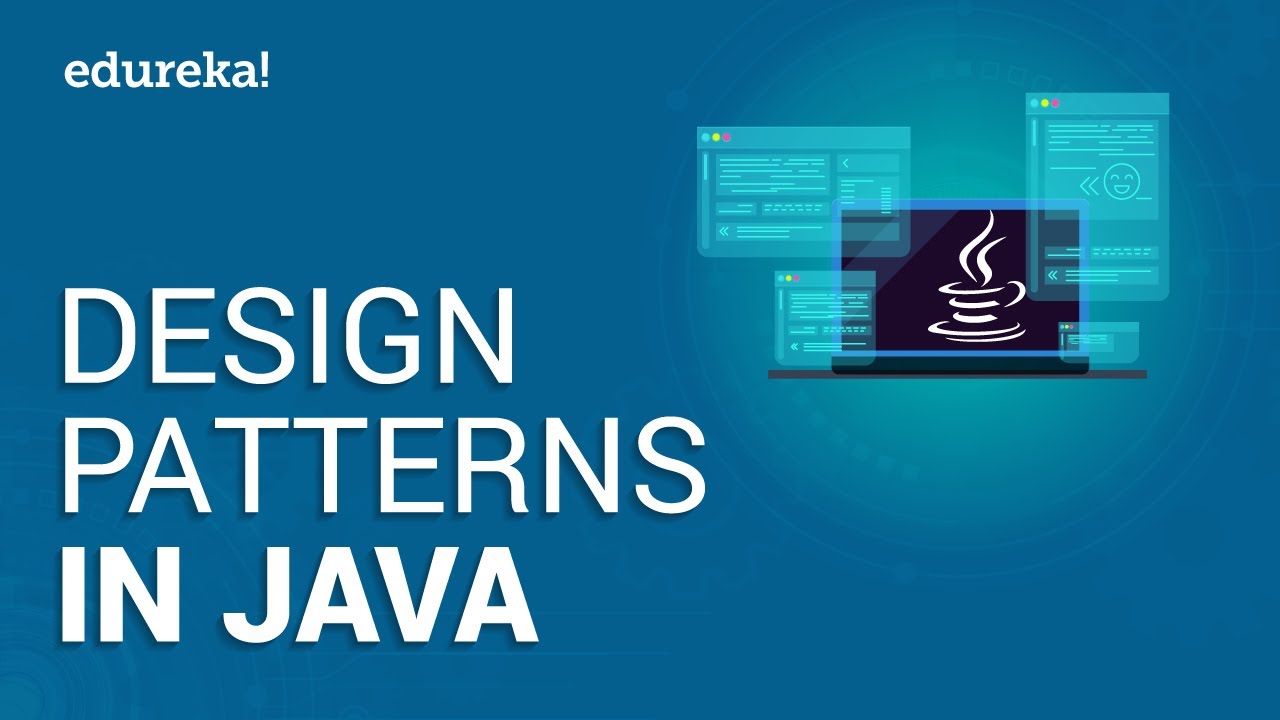
We need to keep in mind that design patterns are programming language independent for solving the common object-oriented design problems. In Other Words, a design pattern represents an idea, not a particular implementation. Using design patterns you can make your code more flexible, reusable, and maintainable. The Template Method pattern defines the skeleton of an algorithm in an operation, deferring some steps to subclasses. It lets subclasses redefine certain steps of an algorithm without changing the algorithm's structure. It's useful when you want to share common behavior between classes with different implementations.
So when an exception occurs in the try block, it’s sent to the first catch block to process. If the catch block is not able to process it, it forwards the request to the next Object in the chain (i.e., the next catch block). If even the last catch block is not able to process it, the exception is thrown outside of the chain to the calling program.
A design pattern can be reused in multiple applications, and that is the main advantage of using it. It can also be seen as a template for how to solve a problem that can occur in many different situations and/or applications. It is not code reuse as it usually does not specify code, but code can be easily created from a design pattern. Object-oriented design patterns typically show relationships and interactions between classes or objects, without specifying the final application classes or objects that are involved. They are best practices or templates that developers can use to solve problems that occur repeatedly in software development.
However, this will lead to the create(AccountType type) method becoming increasingly bloated. The first strength of the Factory pattern that we can see is that it centralizes the creation of objects in one place. It knows which building steps to call to produce this or that car model. Let’s consider a simplified scenario where you have a shopping cart containing different types of products, and you want to apply different types of discounts to the products in the cart. The Visitor Pattern can help you achieve this by allowing you to define discount calculation logic in separate visitor classes. Using design patterns speeds up your design and helps to communicate your design to other team members.
The Factory Method pattern defines an interface for creating an object, but the specific class instantiation is deferred to subclasses. Abstract Factory patterns work around a super-factory which creates other factories. This type of design pattern comes under creational pattern as this pattern provides one of the best ways to create an object. We're going to create a Shape interface and concrete classes implementing the Shape interface. We will then create an abstract decorator class ShapeDecorator implementing the Shape interface and having Shape object as its instance variable.
The mediator pattern focuses on providing a mediator between objects for communication and implementing loose-coupling between objects. The mediator works as a router between objects, and it can have its own logic to provide a way of communication. Template Method is a Behavioral Design Pattern, it defines the skeleton of an algorithm in a method but lets subclasses alter some steps of that algorithm without changing its structure. Behavioral design patterns are concerned with algorithms and the assignment of responsibilities between objects.
The flyweight design pattern is used when we need to create a lot of Objects of a Class. Lets you define a subscription mechanism to notify multiple objects about any events that happen to the object they're observing. A proxy controls access to the original object, allowing you to perform something either before or after the request gets through to the original object. Lets you split a large class or a set of closely related classes into two separate hierarchies—abstraction and implementation—which can be developed independently of each other.
No comments:
Post a Comment